This is the lecture note of CS61A - Lecture 1.
What is Computer Science?
Computer Science has many subfields, and each subfield has its own sub-subfield.
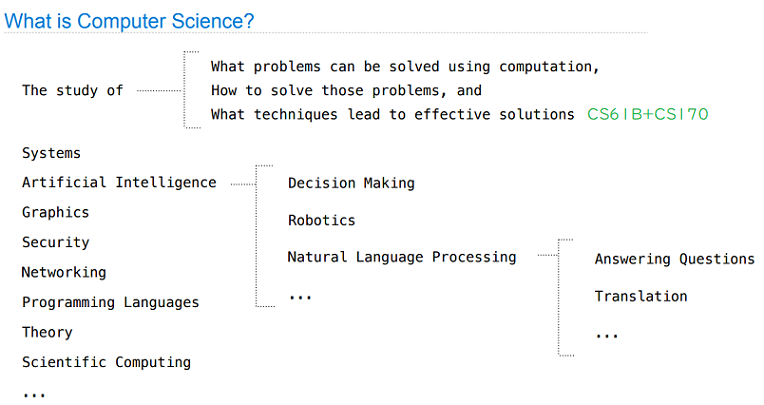
What is this course about?
- A course about managing complexity, thus you should:
- master abstraction
- procedural abstractions
- data abstractions
- master programming paradigms
- master abstraction
- An introduction to programming:
- full understanding of Python fundamentals
- combine multiple ideas in large projects
- learn how computer interpret programming languages
- Different types of languages:
- Python
- Scheme
- SQL
Demo
1 | # Numeric expressions |