This is the lecture note of CS61A - Lecture 2.
Expression
An expression describes a computation and evaluates to a value.
Function, as a generalization over all notations, can be used by all expressions.
1 | """ |
Evaluation of call expression
An expression's operators and operands can also be expressions.
An expression tree is as follows:
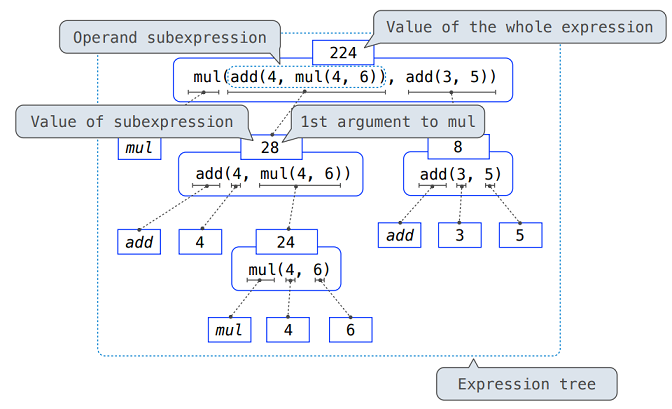
Evaluation procedure for call expressions:
- Evaluate the operator expression
- Evaluate the operand subexpressions from left to right
- Apply the operator (a function) to the operands (arguments)
Names, Assignment, and User-defined Functions
Assignment
Assignment is a simple means of abstraction: binds names to values or functions.
Examples:
1 | from math import pi, sin |
Defining Functions
A function is a sequence of code that perform a particular task and can be reused easily. Function definition is a more powerful means of abstraction.
We programmers can build our own functions.
1 | """Just function definition won't make the function be executed.""" |
1 | # User-defined functions |
Procedure of calling user-defined functions is as follows:
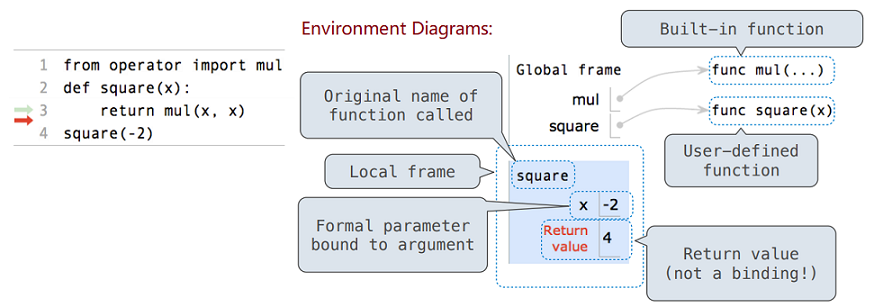
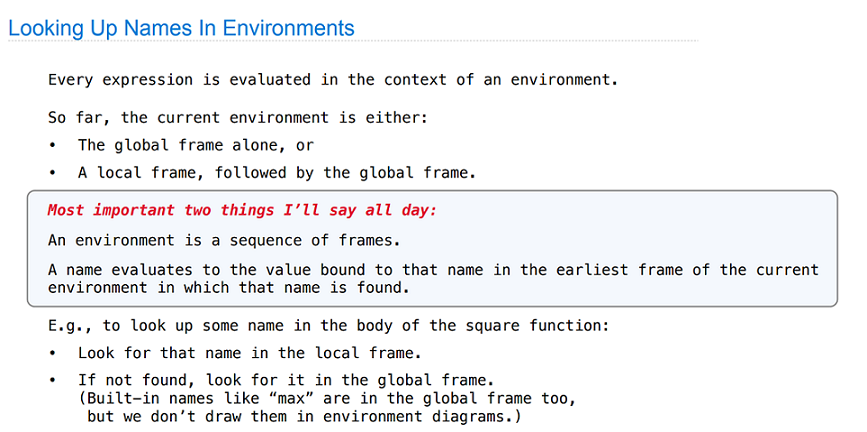
Modules
Functions are organized into modules, which together comprise the Python library. For example, Numpy is a library, it contains many modules, i.e. many .py
files.
We programmer can define our own functions modules or libraries.
🦄 External Resource: Python Standard Library