This is the lecture note of CS61A - Lecture 4.
Iteration Example
There are 2 different definitions of fib
which can be used to calculate Fibonacci number.
1 | def fib(n): |
The second one is much better since it can computer the 0-th Fibonacci number correctly.
Designing Functions
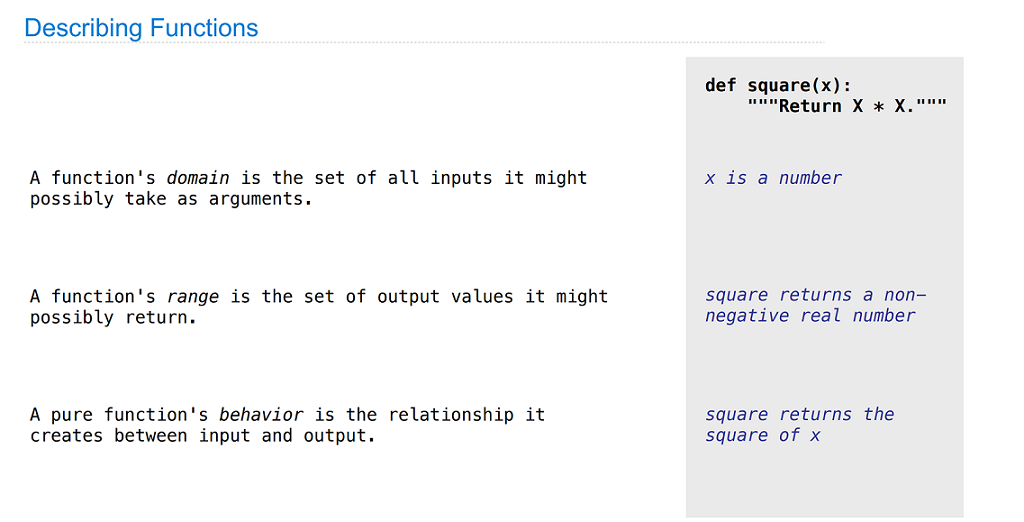
There are lots of different functions that can do the same thing, but some are better than others.
So, how to design better function?
🏜️ A Guide to Designing Function:
- Give each function exactly one job.
- Don’t repeat yourself (DRY). Implement a process just once, but execute it many times.
- Define functions generally.
Example
The following is an example of generalizing patterns with arguments.
1 | # Compute the area of square, circle and hexoagon |
Noticing there are lots of repeating things among them.
Let's try to do some generalization.
1 | # Generalization: Generalizing patterns using arguments |
Higher-Order Function
The common structure among functions may be a computational process, rather than just a number we saw in the previous example.
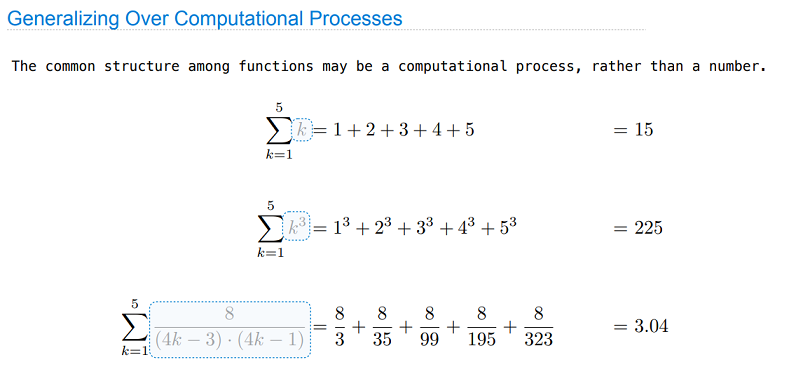
1 | # Without generalization |
We notice the above computational processes are similar. The only difference is how we deal with the value of k-th term.
Let's do generalization again.
1 | # Functions as arguments |
The function summation(n, term)
above is called higher-order function, since it takes another function term
as an argument.
Higher-order functions can also treat functions as return values. For example:
1 | def make_adder(n): |
Higher-order functions :
- express general methods of computation;
- remove repetition from programs;
- separate concerns among functions (each function just do one job)
Lambda Expression
Lambda expression is an expression that evalutes to a function.
1 | # Lambda expressions |
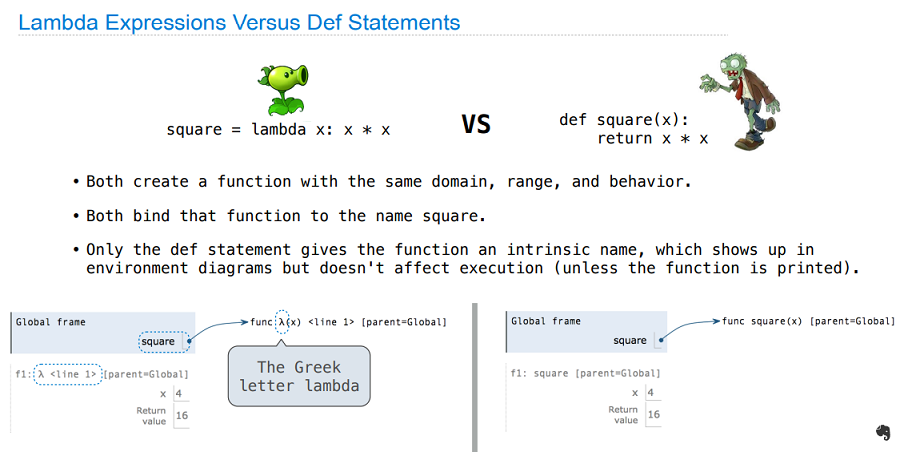
Return Statements
A return statement completes the evaluation of a call expression and provides its value.
Let's look at 2 problems.
Question 1: find the smallest non-negative integer x which makes square(x) - 100 is positive
1 | def search(f): |
Question 2: define inverse function
1 | def inverse(f): |