This is the lecture note of CS61A - Lecture 5.
Environments for Higher-Order Functions
Review: Higher-order function is a function that takes a function as an argument value or returns a function as a return value.
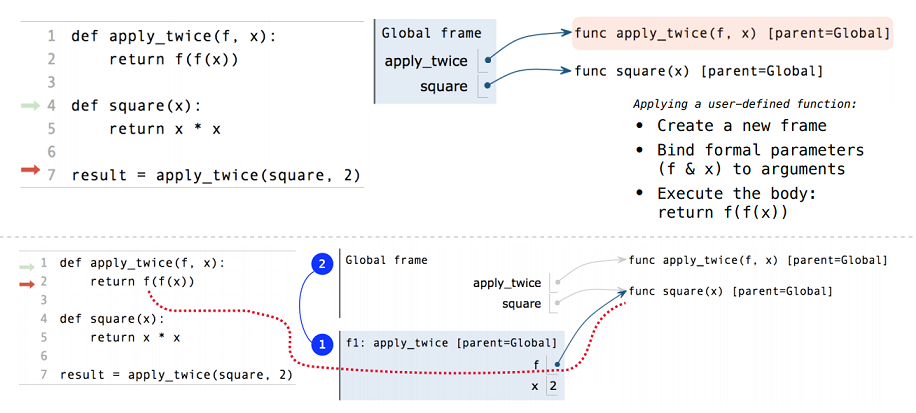
Environments for Nested Definitions
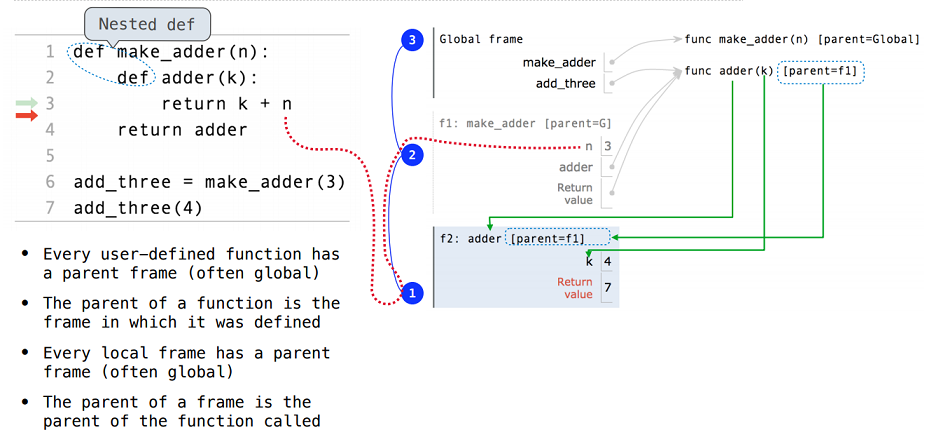
Self-reference
1 | # Self Reference |
Currying
Currying: Transforming a multi-argument function into a higher-order function with single-argument.
1 | # Currying |