This is the lecture note of CS61A - Lecture 8.
Recursive Function
A function is called recursive
if the body of that function calls itself, either directly or indirectly.
Let's see an example.
1 | # Sum digits |
Another example:
1 | # String reversal |
Recursion in Environment Diagrams
Notice: The recursion environment diagram is shown here to help you understand the recursive function execution process. When dealing with the real problems, you should avoid draw this environment diagrams.
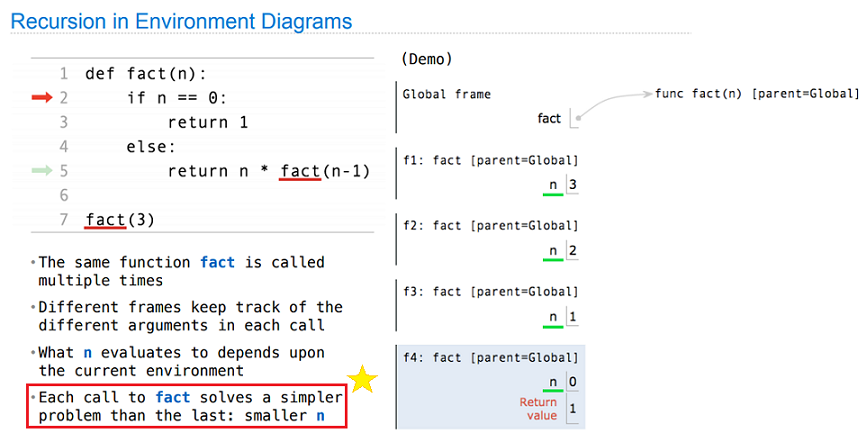
Recursion and Iteration
Iteration is a special case of recursion. For the most of functions, you can convert from iteration into recursion, or vice versa.
Let's see another example.
1 | # Converting iteration to recursion |
Mutual Recursion
Mutual recursion occurs when two different functions call each other.
Let's see an example.
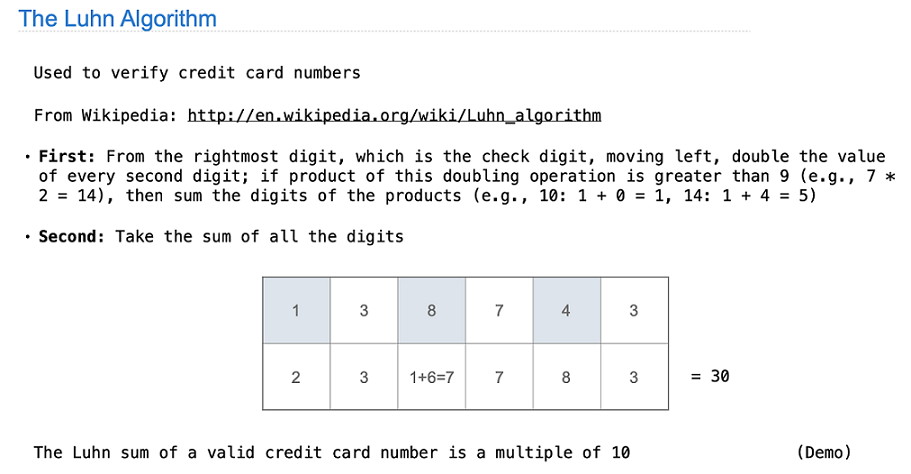
1 | # Luhn algorithm: mutual recursion |