This is the lecture note of CS61A - Lecture 7.
Order of Recursive Calls
When making a function called, you have to wait for return before doing anything else.
Let's see an example.
1 | # Ordering |
- If two implementations are equally clear, then shorter is usually better.
- In this case, the longer implementation is more clear (at least to me).
- When learning to write recursive functions, put the base cases first.
- Both are recursive functions, even though only the first one has typical structure
Exercise : Write a function that prints an inverse cascade.
1 | # Inverse Cascade |
Tree Recursion
Tree-shaped processes arise whenever executing the body of a recursive function makes more than one recursive call.
Let's see an example :
1 | # Tree recursion |
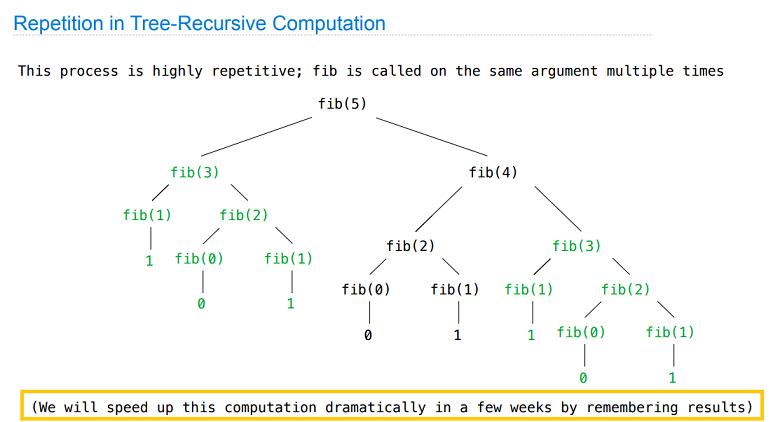
Hanoi Tower
The Hanoi Tower Problem is a very classical recursion problem.
1 | # Hanoi Tower |
Example: Counting Partitions
Let's see an important example of tree recursion —— counting partitions of an integer.
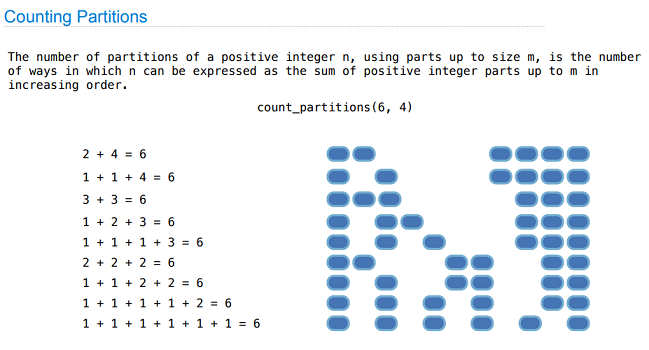
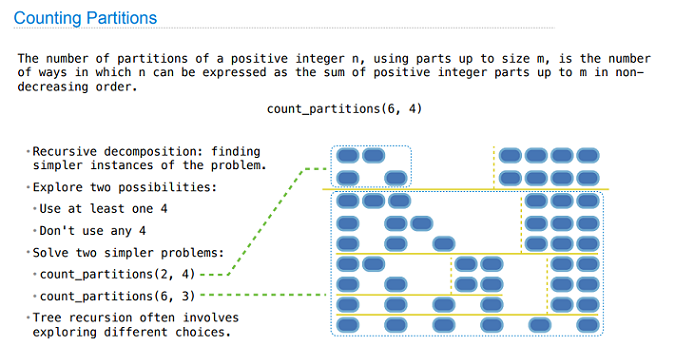
1 | def count_partitions(n, m): |