This is the lecture note of CS61B - Lecture 4.
In this lecture, we will learn about primitive types and reference types in Java, and use these concepts to construct our first data strucure - Linked Data Structures.
Q1: What's your answer of the following question? Will the change to b
affects a
?
1 | /** |
The answer is yes! And the output is as follows:
1 | weight: 5, tusk size: 8.30 |
Q2: Think about another question, will the change to x
affect y
?
1 | int x = 5; |
The answer is no, and x is 2 and y is 5.
You may wonder why. To clear up your confusion, let's explore data types in Java world.
Primitive Types
Your computer stores information in memory. And whatever the information you want to store, ultimately it will be encoded as a sequence of ones and zeros.
Actually, sometimes, two different things will be stored as the same bits sequence. For example, number 72
stored as 01001000, and letter H
stored as 01001000, too.
So how computer interprets them correctly? The answer is the data type tells Java interpreter how to interpret it.
There are 8 primitive types in Java: byte, short, int, long, float, double, boolean, char.
Reference Types
We already know that there are 8 primitive types in Java. Everything else, including array and string, is a reference type.
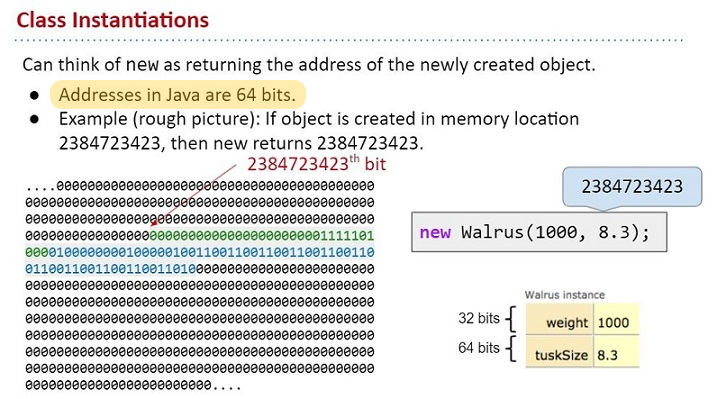
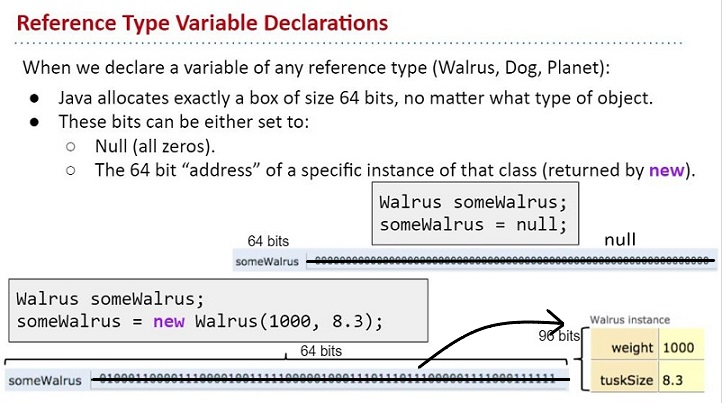
The Golden Rule of Equals
This is a very important rule and can help us answer the above puzzles.
- Given variables x and y, then
y = x
means copies all bits from x into y.
And reference types also obey this rule. If x and y are reference types, after y = x
, x and y will refer to the same object.
Exercise
Try to do the following exercise and make sure you understand everyting so far.
1 | // Does the call to doStuff(walrus, x) have an affect on walrus and/or main’s x? |
The answer is "walrus will lose 100 lbs, but main’s x will not change".
Linked Data Structures
If you have used Python before, you must be familiar with list. Java has list too.
A list is going to be able to grow arbitrarily large, which is different from array. But you may wonder why list can have arbitrary size. To answer this question, you need to know its data structure under the hood, i.e. Linked List.
We will learn it by building it from scratch. Our goal is to build a linked list as follows,
Create Linked List
You can build it intuitively, even though it is awkward.
1 | public class IntList { |
We can make things slightly better by using constructor.
1 | public class IntList { |
Define size( ) Method
In Python, we use len(list)
to get the size of list. In Java, we use L.size()
. How can we implement it?
1 | /** Return the size of the list using ... recursion! */ |
Define get( ) Method
1 | /** Return the i-th item of the list using ... recursion! */ |
Final code
The following is the overall code we built in this lecture. It reveals the structure of linked list although it is hard to use. In the next lecture, we will improve our implementation of linked list. See you next time 🤠
1 | public class IntList { |