This is the lecture note of CS61B - Lecture 9.
🌳 In this lecture, we will talk more about implementation inheritance.
Implementation Inheritance: Extends
In the last lecture, we talked about implements keyword. When a class is a hyponym of an interface, we use implements.
If you want one class to be a hyponym of another class, you use extends.
1 | /* SLList, but with additional rotateRight operation. */ |
We should notice that constructors won't be inherited.
Let's look at another example. Suppose we want to build an SLList called VengefulSLList that:
- Remembers all Items that have been destroyed by
removeLast
. - Has an additional method
printLostItems()
, which prints all deleted items.
1 | public class VengefulSLList { |
How should we do?
1 | /** SList with additional operation printLostItems() which prints all items |
Constructor Behavior
❗ Important Note: both implements
and extends
should only be used for is-a relationship instead of has-a relationship.
Encapsulation
Module: A set of methods that work together as a whole to perform some task or set of related tasks.
A module is said to be encapsulated if its implementation is completely hidden, and it can be accessed only through a documented interface.
🌴 Reminder: You should use the idea of encapsulation to guide yourself when completing project1b, and other large project.
Type Checking and Casting
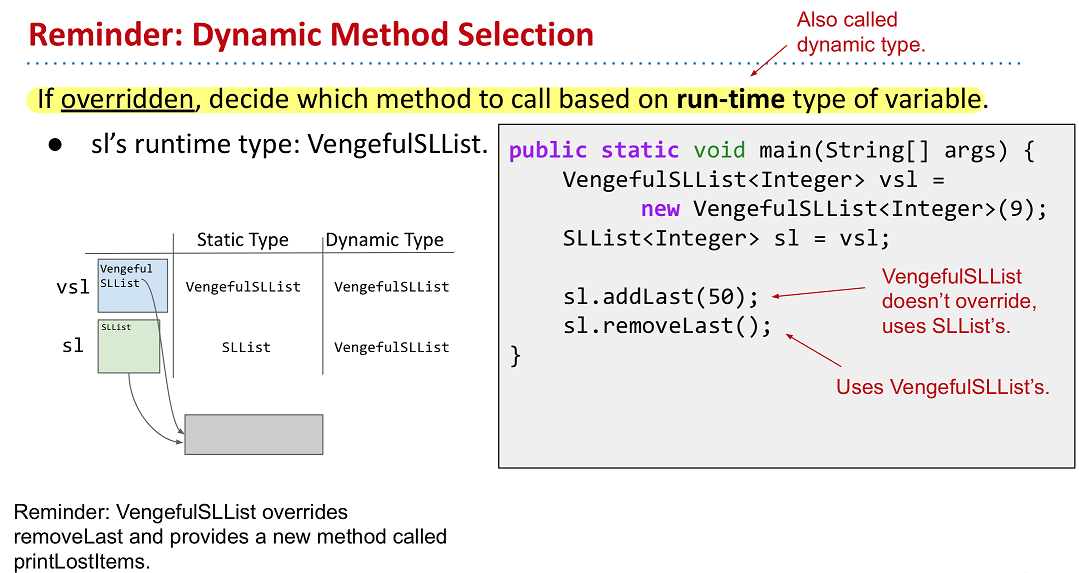
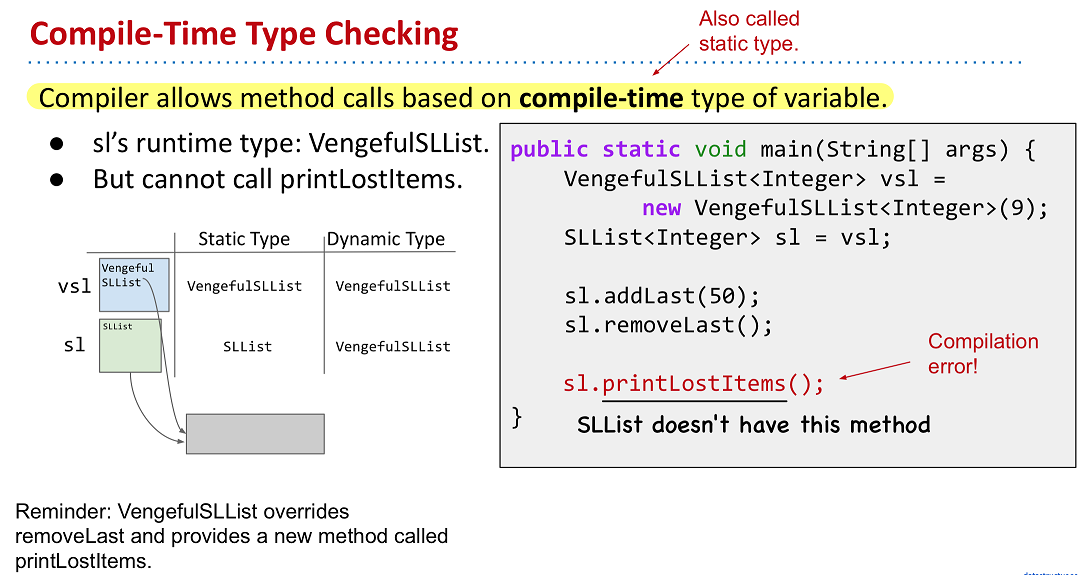
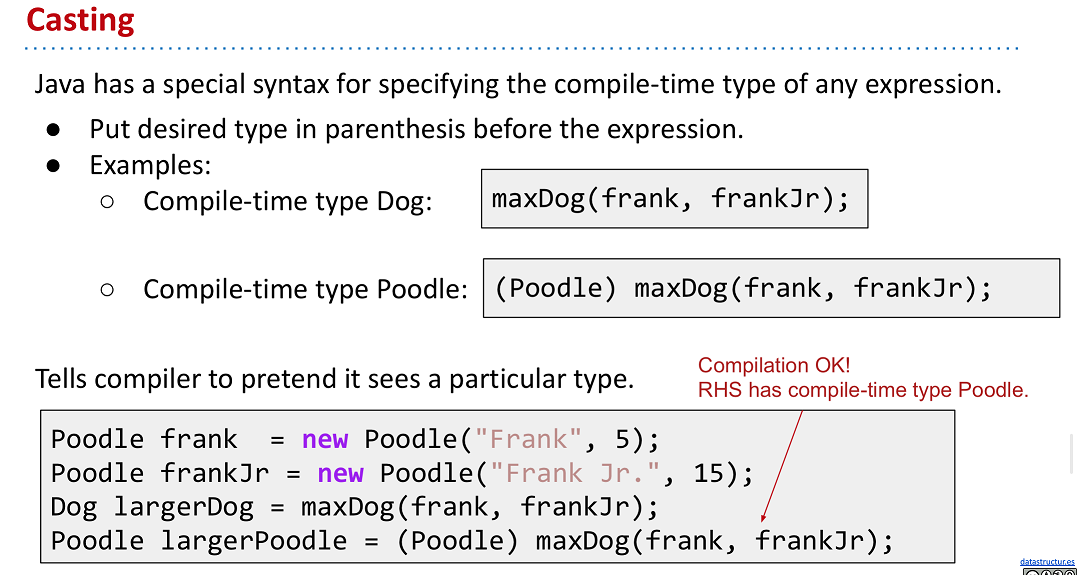
Higher Order Functions
Python is good at defining higher order functions (HOFs).
1 | # example of HoF |
HoFs is powerful and useful. However, before Java7 and earlier, there is a fundamental issue that memory boxes(variable) cannot contain pointers to functions. So if you want to define HoFs in Java7 or earlier, you should use interface instead.
Let's see an example.
1 | /** Represent a function that takes in an integer, and returns an integer. */ |
1 | public class TenX implements IntUnaryFunction { |
1 | public class HoFDemo { |
HoFs in Java8 or Later
In Java 8, new types were introduced, and you can hold references to methods.
1 | import java.util.function.Function; |